Solidity Programming and Ethereum Smart Contracts
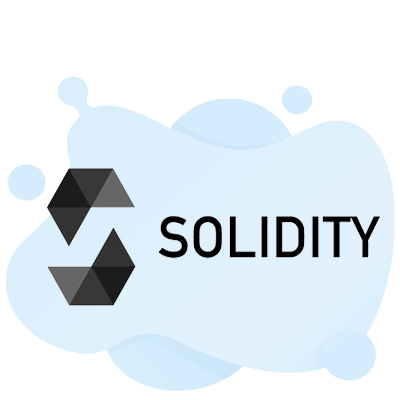
Solidity is an object-oriented programming language that is used to create smart contracts on the Ethereum blockchain. It was created by Christian Reitwiessner and Alex Beregszaszi, and programs written in this language run on the Ethereum Virtual Machine. You can learn more about it in this article. In addition to its use in Ethereum smart contracts, Solidity is also used in many other projects.
Free Solidity Practice Test Online
Solidity Web Dev Questions and Answers
Solidity is an object-oriented programming language to implement smart contracts on different blockchain platforms, most notably Ethereum.
Examining some of the online resources is one way to start learning Solidity. On the Solidity website, for instance, there are a few introductory tutorials. On the Ethereum Developer Wiki, you can also find documentation and code examples. Attending a workshop or training course is an additional choice. Several organizations provide these courses, but they differ in terms of price and content. Finally, reading the Solidity Language Reference may be a more effective way to learn Solidity if you are already familiar with programming languages.
It may take one to six months to complete.
Even beginners without prior experience can pick it up following a predetermined training program because it’s relatively simple to learn.
A solidity developer who works with smart contracts on Ethereum-based applications is known as a Solidity developer.
There are several ways to learn Solidity development. You can read books or tutorials, take an in-person or online training course, enroll in a coding bootcamp, or all three. No matter your path, having a solid foundation in Python and programming theory is critical. After establishing that base, you can begin learning about Solidity’s specific features. Do some research; there are plenty of printed and online resources available.
Ethereum Network team specifically developed Solidity, an object-oriented programming language, for building and designing intelligent contracts on Blockchain platforms. The blockchain system is used to create smart contracts that carry out business logic and produce a string of transaction records.
- Open Remix IDE in any of your browsers, click New File, select Solidity as the environment, and then click OK.
- Write Smart contract in the code area, and then compile the contract by clicking the Compile button in the Compiler window.
- brew install node.
- npm install -g solc.
- brew tap ethereum/ethereum.
- brew install solidity.
- Knowledge of the basics of blockchain.
- Knowledge of the Ethereum platform
- Prior knowledge of any programming language, such as JavaScript, C, or Python.
- Basic understanding of programming principles.
- Knowledge of the command line is required to create new directories.
There are 200,000 Solidity developers.
In Solidity, calling a function is as simple as writing its name in the appropriate place. When calling a function, different parameters can be passed, and multiple parameters can be passed by separating them with commas.
Utilizing the Truffle console is the most effective way to determine the Solidity version. Change directories (cd) to your project folder in a terminal after opening it. Then enter after typing truffle console.
Click the Solidity compiler button to compile your code. The names of the buttons on the left side of the editor should be visible when you hover over them. Go ahead and click the Compile helloWorld button.
These steps will help you use Solidity to create a smart contract:
- Decide on a language.
- Start a new project.
- Import the necessary libraries.
- Compose some code.
- Build your program.
- Implement the contract
- The procedure is relatively simple if a deployment script is being used. Make a file with the bytecode for your contract first. Run the script after that using the private key for your account and the address of the Ethereum node you want to deploy to.
- The procedure is trickier if you’re using a standalone Ethereum client. However, the majority of clients offer a platform for deploying contracts. For instance, in Geth, arrangements can be deployed using the “deploy” command.
- Turn on Developer Mode in your computer’s settings if it is not already enabled. To accomplish this, go to “Settings,” which is indicated by the gear icon in the “Start” menu. Now look for “Developer settings” in the “Settings” window’s search bar.
- The Windows Linux Subsystem feature should be enabled. To do this, use the “Start” menu search box to look up Windows features.
- Make a directory to house all of the Solidity-related work you’ll be doing. You can create this in the desktop directory by using the name solidityProject.
- Install each dependency that is required. You can run Solidity on your local computer using a variety of techniques. We’ll employ NodeJs. Use the following command to install cURL in your Bash environment.
- Master Ethereum & Solidity Programming From Scratch in 2023 [Udemy]
- Certified Solidity Developer [Blockchain Council]
- The Complete Solidity Course – Blockchain – Zero to Expert [Udemy]
- Learn Solidity: Programming Language for Ethereum Smart Contracts [Blockchain Council]
Solidity does not support printing. Only the console can be used for writing.
- Print directly to the blockchain using the console: (“Hello, world!”) web3.toWei. send()
- To print, use the tokens.print function from the ERC20 Token Standard Library ()
- Use the function externalContract.print to print an external contract ()
Ctrl+Shift+P will launch the command palette in Visual Studio Code. Then click “compile current Solidity contract” after typing “solidity”.
To verify and publish the contract, click the blue “Verify and Publish” button after pasting the contract’s source code into the input box labeled “Enter the Solidity Contract Code below.” The green checkmark ought to now be visible on the Contract tab. The contract can directly be interacted with because it has been verified.
When a new language version is released, the Solidity compiler is automatically updated. You don’t need to take any action.
contract SafeMath { function safeMul(uint a, uint b) internal returns (uint) { //returns the safe product of a and b //simplifies multiplication by avoiding overflow uint c = a * b; //c will be between 0 and uint256_MAX if (c > uint256_MAX) c = uint256_MAX; //overflow protection else if (c < 0)
Yes. The Ethereum blockchain uses the programming language Solidity to create smart contracts. Although other languages can be used for this, Solidity is by far the most well-liked and common.
The sender of a message is the address that called or initiated a function or created a transaction.
Someone can send ether to a contract and run code to account for this deposit using the keyword payable.
The curly-bracket language Solidity is intended to work with the Ethereum Virtual Machine (EVM). JavaScript, Python, and C++ influence it.
You can create more complex data types with multiple properties using Solidity’s structures.
Solidity has the most standard control structures and conditional statements found in other programming languages, except the switch and goes to control structures.
Gavin Wood
Mastering Solidity can create Smart Contracts (A key blockchain component). Learning Solidity is necessary only if you want to create smart contracts.
There is not a lot of demand for Solidity developers. However, as the markets look for their programming skills to develop new projects, their order will gradually peak.
It might take longer if you have no prior programming experience.
Solidity is the language needed to create a BSC token.
Solidity is not the programming language used by Solana.
Globally, a Solidity Developer makes $99,110 a year on average.
The simplest method is to call it such as A. foo(x, y, z).
There are several ways to profit from Solidity. Making dapps and charging for their use is one approach. Another option is to create smart contracts for clients and charge them for your services. Additionally, you could write tutorials or articles on Solidity to aid others in learning the language and earn money. Finally, you could create and market software libraries or tools that help coding or deployment. There are many options, so look into what interests you and move forward.
Since Solidity arrays are indexed starting at 0, the following code would return an array: return arr[0..5];
IntelliJ Solidity can be used in a variety of ways. Installing IntelliJ and the Solidity plugin is one option. Making use of an online editor like Remix is an additional option. Finally, the Solidity compiler is also available in a standalone version.
- Open Remix IDE in any of your browsers, click New File, select Solidity as the environment, and then click OK.
- After writing the Smart contract in the code section, compile the contract by clicking the Compile button in the Compiler window.
- In the Deploy and Run Transactions window, select the Deploy button to run the code.
- To run the program after the code has been deployed, click on the method calls under the drop-down menu for deployed contracts, and then click on the drop-down on the console to view the output.
- To debug, click the Debug button in the console that corresponds to the method call. Each function call and variable assignment can be verified here.
Learning Solidity in 2023 is definitely worthwhile. The language is used in many well-known blockchain platforms, including Ethereum, EOS, and Tron, and its popularity is only increasing. The need for Solidity developers is rising along with the adoption of blockchain technology. Therefore, learning Solidity is an excellent place to start if you want to enter the blockchain industry.
The curly-bracket language Solidity is intended to work with the Ethereum Virtual Machine (EVM). It is influenced by JavaScript, Python, and C++.
No matter how complex the solidity code is, Solidity Finance is respected in the community and relied upon as a top smart contract auditing company.
They are open source, which is their main difference. Similar to how apps in the Apple app store are developed to run on iOS, dApps on Ethereum are developed to run on Solidity.
Since they are all employed in the development of Dapps, they are all Turing complete.
For those new to programming, it is advised to learn Javascript or Python before learning Solidity. Even though it might take longer, doing this will benefit you in the long run by strengthening your foundation as a developer.
Numerous blockchains employ Solidity as their primary programming language. The most well-known example is Ethereum, but there are numerous other projects that are either in development or have already been released using Solidity. Among these are:
- Akasha
- Bancor
- BlackCoin
- BitShares
- ChronoBank
- Decred
- Ethereum Classic
- Gridcoinclassic
To build and design smart contracts on Blockchain platforms, the Ethereum Network team developed Solidity, an object-oriented programming language.
A Solidity Developer creates and deploys smart contracts on Ethereum-based applications using the object-oriented Solidity language.
The default method of interacting with contracts in the Ethereum ecosystem, both from outside the blockchain and for contract-to-contract communication, is the Contract Application Binary Interface (ABI).
A data type with 20 bytes is an address. It is made specifically to store Ethereum account addresses, which are 20 bytes or 160 bits long.
A contract’s inheritable member, known as an event, records the arguments passed when it is emitted in the transaction logs.
In Solidity, mapping functions similarly to another language’s dictionary or hash table.
On the Ethereum network, the msg. value is a component of the msg (message) object when sending (state-transitioning) transactions.
If the specified condition returns an actual value, “require” returns two boolean values that are either true or false, allowing the code to proceed and work as intended. If the result is false, the code is immediately terminated, and an error is thrown.
Since Solidity was created using existing programming languages like C++, Python, and JavaScript, it uses language structures similar to those found in those languages, making it simple for developers to adopt.
A contract in Solidity is a group of code (its functions) and data (its state) that is stored at a specific address on the Ethereum blockchain. Solidity’s code is contained within contracts.
According to Tang et al. (2019), the solidity ratio (S n) is the proportion of the netting’s projected area (S P) to its outline area (S 0).
August 2014
With the Remix IDE, you can directly test code examples in your browser. Without installing Solidity locally, you can write, deploy, and manage Solidity smart contracts using Remix, a web-based IDE.
The simplest answer is that polygons do not use solidity. A programming language called Solidity was created specifically for creating smart contracts for the Ethereum blockchain. A 3D modeling program called Polygon is used to build and sculpt 3D models. Although they have a few similarities, these two programs are very different and serve different objectives.
You can print logging messages and contract variables when running your contracts and tests on the Hardhat Network by calling console.log() from your Solidity code. You must import hardhat/console.sol into your contract code to use it.
- Find the mapping objects
- Specify the Quantity of ICO Tokens
- Determine the Total Token Supply
- Approve the withdrawal of tokens by delegates.
- Discover how many tokens have been approved for withdrawal.
- Add the Solidity Library for SafeMath.
Solidity doesn’t have a built-in function to produce random numbers. However, you can produce a random number using the blockhash function. Using an uint256 as its input, the blockhash function outputs a 32-byte string. The last few bytes of the returned string can be used as your random number using this function to generate random numbers.
There are numerous solutions. The first one is based on the block’s timestamp: each timestamp should be larger than the parent’s. However, nodes can manipulate timestamps, especially if it promises them profits. The second method is based on using the block numbers as they are ordered one by one and calculating the current time by knowing the average block execution time. Unfortunately, the block mining time may vary, particularly with new Ethereum updates.
You can return multiple values from any function in Solidity. You can accomplish this by either directly assigning the matter to the variable name specified in the returns() statement or using the return(value1, value2,…) statement.
Specify the desired Solidity version in your truffle.js file to update the Solidity version in Truffle. As an example, consider the following: module.exports = { // Other settings here… solium: { presets: [‘latest’], // Change this to set the desired Solidity version solidityVersion: ‘0.4.21’ }, };
Structs can be used in a variety of ways in Solidity. The simplest method is to create a new struct instance and give the values of its fields. The struct keyword can also be used to create a new type, which you can then use as the type of a variable, a function parameter, or a return value.
Solidity generates bytecode, which is interpreted by the Ethereum virtual machine (EVM).
For those involved with and curious about the Solidity language and its surrounding ecosystem, there is a free interactive forum called the Solidity Summit.
Smart contracts can be implemented using Solidity, an object-oriented high-level language.
According to the Solidity documentation, Python is also a significant influence in addition to C++ and JavaScript.
Solidity should be your first choice if your main objective is to start developing blockchains and smart controls.
A modifier is defined as follows in the Solidity documentation: An at-compile-time source code roll-up is a function modifier.
Variables whose values are stored in a contract storage system indefinitely.
When an event is generated, the arguments passed are recorded in transaction logs.
Solidity Storage
Solidity stores state variables in arrays called slots. Each slot has a pre-defined size and is accessed by contract code order. The default size of a storage array is 2256 bytes. It is possible to change the size of the array by setting the length parameter. In this article, we’ll look at the different types of storage and how each works.
The slots in Solidity are the equivalent of the RAM and hard drive of a computer. The only difference is that you don’t have to allocate and release space. This is because the smart contract storage has 2256 locations, roughly the same number as the number of atoms in the universe. Because of this, you can store any number of values on a single smart contract.
Solidity also uses a temporary data location called calldata. A calldata is a memory location that sits in the memory of the EVM. You can use this storage when you don’t need access to the data. But keep in mind that calldata variables are only available within a function’s declaration. Knowing this will prevent you from making silly mistakes.
Why Is A Deployer Used Solidity
When a deployer wants to run code on the blockchain, they can use the Solidity language. This language provides several functions for error handling. For example, it can automatically revert the state of the blockchain when an error occurs. It also prevents unauthorized code access by providing an assert() and require() method.
Solidity Developer
If you are interested in learning how to code in Solidity, you should begin by learning the basics of the language. Ethereum was created by Gavin James Wood, a computer scientist from England. His work has since been used in several projects, including Polkadot, Kusama, and Ethereum.
A Solidity developer can help your business build applications with self-infusing business logic and non-repeatable transactional records. They can also develop smart contracts on the Ethereum or other private Blockchain platforms. Using a hiring funnel is a good way to determine which Solidity developer will work best for your project.
A dedicated Solidity developer can make a world of difference for your business. A dedicated team can help you complete your project efficiently without the need for you to spend a lot of extra money. These developers can also ensure your content is informative and relevant to your audience. All of this means a higher ROI for you.
Solidity Definition
Solidity is a high-level programming language that enables developers to write smart contracts on the Ethereum blockchain. It is similar to JavaScript and is considered a dialect of that language. It also shares similarities with C++ and Python. The most important use of Solidity is for building smart contracts. These contracts automatically control assets and distribute funds when conditions are met.
When used in the proper context, solidity can mean the property of being solid. It also means the quality of being firm and substantial. For instance, solidity refers to the strength and stability of mind, character, and finances. It also refers to the volume of matter that a solid body occupies.
The most fundamental human quality is solidity. A person must have solidity to stand for what they believe in and deliver what they truly feel. The word “solid” comes from the Latin word “nome,” meaning “name.” It defines what things are.
Hardhat Solidity
Hardhat is a dapp development environment for Ethereum. It includes a helpful stack trace, multiple Solidity compiler versions, robust Mainnet fork support, TypeScript support, and contract verification with Etherescan. It is free to sign up for and use. Read on to learn more about it.
Hardhat deploys contracts by the first signer, rather than by the whole contract. Because the contract owners can be specific addresses, it’s not optimal to iterate over all signers. However, it can be used locally. It also offers documentation and a quick installation process. The npx command makes it easy to use Hardhat.
Hardhat works with the Ethereum API to build and deploy smart contracts. It is easy to use and comes with many plug-ins for Ethereum. It also has a Telegram channel for developers to discuss their projects.

Solidity External vs Public
When creating functions in Solidity, there are two types of functions: internal and external. An internal function can only be called internally, while an external function can be called from any contract. Both types of functions use pointers to memory to pass arguments. For an internal function, pointers to memory are passed as arguments to an array, while the arguments to an external function are always passed as local variables.
An external function is a part of the interface of a contract, but cannot be called internally. It promises not to modify the data in the contract. Common examples of an external function are “getter” functions and “constructor” functions. A constructor function executes only once, when the contract is first deployed, but it sets up the state variables, which allow the contract to send ETH to other accounts.
A public function can be called by any contract, while a private function can only be called from the contract in which it was defined. As you can see, each of these modes has their advantages. For example, a public function can be called from an internal contract, while a private function can only be called from another contract.
Solidity Memory Keyword
When creating a solidity program, you need to be aware of how it works with memory. The memory used by Solidity Smart Contracts can be any size, both during execution and between function calls. Memory can also be used to access data stored on Storage. Here are some tips to help you understand Solidity memory.
Solidity has two types of memory: fixed size arrays and dynamic arrays. Arrays can hold different types of elements, depending on their size. You must be careful with the memory array element type. A mapping will not work with a memory array, which is why you must specify the type of array element. An array is an ordered collection of memory locations. The lowest address corresponds to the first element, and the highest address corresponds to the last element. Arrays can be dynamic or compile-time. When creating a memory array, you must follow the Solidity memory management rules.
Solidity supports two different types of memory: reference types and value types. Value types store data directly in memory, while reference types duplicate variables in assignments and functions. This helps keep them independent and ensures that changes made to one variable will not affect the other.
Solidity Remove From Array
The Solidity removes from array function is used to delete one of the elements in an array. Arrays can be either dynamic or compile-time fixed. The removal of an element does not change the length of the array. Instead, a gap is created in the array. Solidity supports infinite arrays.
The delete function removes elements from an array by shifting them to the left or right. You can also use the reducing length property to delete elements from the array. This method has some additional benefits. It also behaves differently than the other methods: it permanently destroys named elements and element properties. When deleting elements from an array, you should use this function carefully.