Scala Programming Language 2023
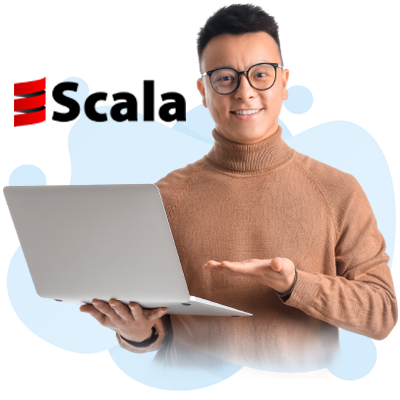
Scala is a high-level language that mixes object-oriented and functional programming. Scala’s static types aid in the avoidance of problems in complicated applications, and its JVM and JavaScript runtimes enable the development of high-performance systems with simple access to vast ecosystems of libraries. Scala source code can be compiled into Java bytecode and executed on a Java virtual machine (JVM). Scala and Java share language compatibility, allowing libraries in either language to be referenced directly in Scala or Java programs. Scala, like Java, is object-oriented and employs a syntax known as curly-brace, which is comparable to the language C. Since Scala 3, the option of using the off-side rule (indenting) to organize blocks is recommended. According to Martin Odersky, this was the most fruitful feature introduced in Scala 3.
Scala is a combination of scalable and language, indicating that it is intended to evolve in tandem with the needs of its users. Unlike Java, Scala has numerous functional programming language characteristics, such as currying, immutability, lazy evaluation, and pattern matching. It also features a sophisticated type system that supports algebraic data types, covariance and contravariance, higher-order (but not higher-rank) types, and anonymous types. Scala also has operator overloading, optional arguments, named parameters, and raw strings, which Java does not have. Checked exceptions, which are not available in Scala, are a controversial feature of Java.
Scala is a programming language used in data processing, distributed computing, and web development. It underpins many companies’ data engineering infrastructure. It is intended to scale to meet the needs of its users, from developing simple scripts to constructing a large data processing system. Software developers and data engineers mostly use Scala. Some data scientists will use it alongside Apache Spark to process large amounts of data. Scala frameworks that are popular include:
- Play – It is used in the development of web applications.
- Spark – Popular data processing framework.
- Lift – a web framework
Scala for Loop
For loops are also known as for-comprehensions in Scala. A for loop is a repetition control structure that allows us to create a loop that will be executed several times. The loop allows us to complete an n number of steps in a single line.
Syntax:
for(var x <- range){
// Code..
}
The Range could be a number range represented as i to j or sometimes as i until j. The left-arrow operator is a generator because it generates individual values from a range.
Scala List
The Scala List is a linked list that implements an immutable sequence of entries. A linked list, unlike an array, is made up of many small items, each of which contains a reference to another object and the remainder of the list. Lists allow for the quick inclusion of additional elements at the top. To begin, lists are immutable; meaning list components cannot be modified by assignment. Second, whereas arrays are flat, lists represent a linked list. Here are some key points concerning lists in Scala:
- The Scala package scala.collection.immutable defines list.
- Each entry in a Scala list must be of the same type.
- A List has many methods to add, prepend, max, min, and so on to improve its usability.
- During list building, the implementation takes advantage of mutable state.
Scala List definition syntax:
- val variable_name: List[type] = List(item1, item2, item3)
- val variable_name = List(item1, item2, item3)
Scala Array
In Scala, an array is a type of collection. The array data structure in Scala maintains a fixed-size sequential collection of elements of the same type. A collection of data is stored in an array, although it is generally more convenient to conceive an array as a collection of variables of the same type. The index of the first array element is zero, and the index of the last array element is the total number of elements minus one. It is a set of adjustable values. It correlates to arrays in java in terms of syntax, but it differs in terms of functionality from java.
Scala Array types include:
- Single dimensional array
- Used to keep elements in sequential order Array items are kept in a single block of memory.
- Multidimensional array
- An array that stores data as a matrix. Depending on your needs, you can generate arrays ranging from two to three, four, and many more dimensions.
Syntax:
- var z:Array[String] = new Array[String](3)
- var z = new Array[String](3)
Scala FoldLeft
The foldLeft() method is a TraversableOnce trait member used to collapse collections’ elements. It moves elements from left to right. It is typically utilized in recursive procedures to prevent stack overflow errors. FoldLeft works with both Scala’s Mutable and Immutable collection data structures. The foldLeft method accepts an associative binary operator function as an argument and uses it to collapse collection elements. The traversal of the elements in the collection is done from left to right, hence the term foldLeft. You can also specify an initial value with the foldLeft method.
Â
Syntax:
def foldLeft[B](z: B)(op: (B, A) ? B): B
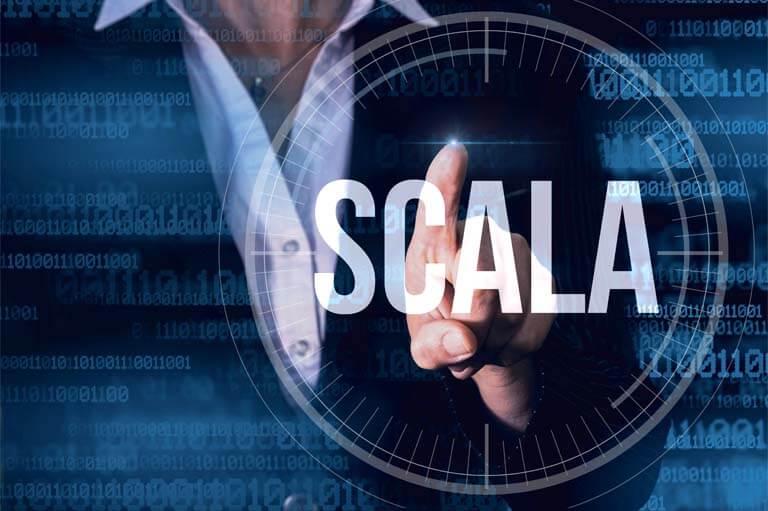
Scala Jobs
Scala is an excellent language to master before diving into big data and data science. Scala’s capability aids its survival in the IT sector. Big data and data science are two emerging fields with a strong need for technical professionals.
Scala Job Descriptions:
- Software Engineer
- Scala Developer
- Scala Programming Language Application Lead
- Senior Software Engineer
- Junior Scala Research Engineer
- Big Data Engineer
- Application Developer
- IT Consultant
String to Int Scala
There is a large library in Scala that supports many string operations. In Scala, one such process is transforming a string into an int. In Scala, use the toInt function, which is accessible on String objects, to convert a String to an Int. This will return the string’s integer conversion. If the string does not contain an integer, an exception with the name NumberFormatException will be thrown.
Syntax:Â
string.toInt
Scala Enum
Enumerations are a language feature that is particularly effective for modeling a limited number of entities. One common example is to model the weekdays as an enumeration, with one value for each of the seven days. Like many other programming languages, Scala has a native mechanism for representing enumerations.
An enumeration is a collection of named constants. To construct and retrieve enumerations, Scala provides an abstract class named Enumeration. Enumerations are used in programming languages to express a collection of named constants. For enumerations, see Enumeration (or enum) in C and enum in Java. Scala includes an Enumeration class that we can extend to create our own enumerations. Enum’s key points are as follows:
- Many functions were inherited when we extended the Enumeration class. Identification is one of them.
- Scala includes an Enumeration class that we can extend to create our enumerations.
- Every Enumeration constant represents an Enumeration object.
- Unlike Java or C, there is no enum keyword in Scala.
- The evaluation’s val members are defined as enumeration values.
Scala foreach
The foreach() method applies the specified function to all of the map’s elements. A foreach loop iterates over the members of an object/container. To traverse list elements, Scala provides the foreach() function. To iterate the list members, Scala provides a built-in foreach() function in the List class, so we no longer need to utilize a loop. This function accepts a lambda expression as an argument and returns the result.
Definition of Method:
def foreach(f: ((A, B)) => Unit): Unit
def foreach(f:(A)⇒Unit):Unit
Scala Mutable Set
Immutable sets are provided by default in Scala. However, we can make our set mutable by simply importing certain packages. Sets are used for storing and retrieving data and only include unique elements; if we try to add duplicate elements, it will discard those items. A mutable collection may be updated or extended in place. As a result, we can add, update, or remove elements from a collection. These collections have operations that alter the collection while it is in place. The scala.collection.mutable package contains all mutable collection classes.
Scala 3 TypeClass
A type class is a parameterized abstract type that allows you to add additional behavior to any closed data type without using subtyping. If you’re coming from Java, type classes are something like java.util.Comparator[T]. Type classes in Scala 3 are just characteristics with one or more type parameters.
This can be useful in a variety of situations, such as:
- describing how a type that you do not own (from a standard or third-party library) complies with such behavior
- expressing such behavior for numerous types without involving sub-typing relationships (one extends another) among those kinds (see: ad hoc polymorphism for instance)
Scala flatMap
The flatMap() function in Scala is equivalent to the map() method in that the inner grouping of an item is eliminated, and a sequence is formed. It is a hybrid of the map method and the flatten method. The result of running the map method followed by the flatten method is the same as running the flatMap method (). To get the required result, flatMap first calls the map method and then the flatten method. When the Scala flatmap method constructs a sequence, it will break the input grouping. We can’t say it’s a combination of map and flatten because it runs both methods consecutively, the first map, then flatten.
Syntax:
defflatMap[B](f: (A) =>IterableOnce[B]): List[B]
Append to Sequence Scala
The list and list buffer can be appended with values. Let’s start with the basics of using Scala’s common methods to add to Scala list and ListBuffer. Use the following techniques to append or prepend one or more elements to a Vector or Seq:
- Use + to append one item.
- Use ++ to append multiple elements.
- To add one thing, use +.
- Use ++ to prepend multiple items.
Scala Case Class Copy
A Case Class is a standard class with the addition of a capability for modeling unchangeable data. It is also beneficial in pattern matching. It has been specified with a modifier case; as a result of this case keyword, we can receive certain benefits to avoid doing sections of code that must be included in many places with little or no modification. The Scala compiler additionally adds a copy() function to the case class, which is used to produce a replica of the same object with or without modifying some parameters.
Scala Question and Answers
In 2018, the TIOBE ranking placed Scala as the 20th most popular language. Despite being ranked No. 14 on Redmonk, it is now lower. Scala serves businesses with valuations in the hundreds of billions, according to Oliver White, chief editor at Lightbend, the firm that created it.
Scala is the same. Scala may be swiftly learned, nevertheless, just like any other programming language. A typical complaint about (learning) Scala is that it has too many features and is difficult for people to link them all, according to my experience teaching 41000+ individuals online at Rock the JVM.
One of the top programming languages for functional programming right now is Scala (it does although still provide support for object-oriented programming approaches). Scala’s popularity in Google searches appears to have peaked between 2018 and 2019.
It is worthwhile to learn Scala. Although Scala is regarded as a difficult programming language, its advantages cannot be disputed. You’ll simultaneously study two paradigms for programming languages. You can work more effectively and productively thanks to Scala’s extensive feature set.
An expert in developing, building, and maintaining Scala-based applications is known as a “Scala developer.” Additionally, they perform software analysis, write code in accordance with app specifications, and work together with the software development team to validate application designs.
Programming in Scala is used to create robust static systems and functional programs. It utilizes the JVM and is object-oriented. It offers the ability to work with Java libraries and existing code. It lacks a concept of primitive data and is widely regarded as a static type language.
Because of its precise grammar, Scala reduces boilerplate code. In comparison to Java-based counterparts, Scala programs need less code. It is both a functional language and an object-oriented language. Scala is the ideal choice for web development because of this combination.
A general-purpose computer language called Scala allows both object-oriented and functional programming on a more extensive level.
One succinct, high-level language called Scala mixes functional and object-oriented programming. Static types in Scala help prevent errors in complicated applications, and the JVM and JavaScript runtimes enable you to easily access vast ecosystems of libraries for developing high-performance systems.
Every function in Scala is a value, making it a functional language as well. Scala has a simple syntax for defining anonymous functions, along with support for higher-order functions, function nesting, and currying.