Benefits of Pattern Design 2023 in Software Development
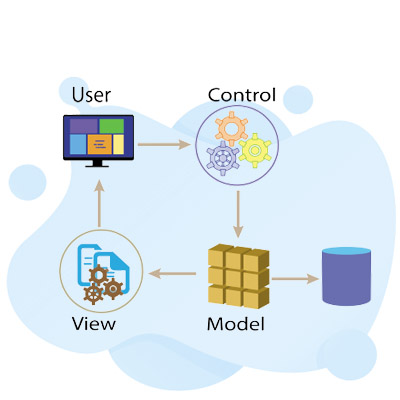
Pattern Design involves defining a design pattern that is used repeatedly to solve a problem. These patterns can be represented visually with class diagrams or interaction diagrams. In the design diagram, participants are listed according to their roles in the pattern. The roles are collaboration, consequences, and implementation. The collaboration part of a pattern defines how classes and objects interact with one another. The implementation part is the part of the pattern that implements the solution.
Free Pattern Design Practice Test Online
Pattern Design Question and Answers
Yes. The MVC design pattern divides an application into three primary logical components: the model, the view, and the controller. Each of these components is designed to handle particular parts of an application’s development.
Design patterns are guidelines on how to solve typical challenges encountered by software developers. They are written in a particular language that developers understand and give a framework for issue resolution that can be utilized in a variety of contexts.
In Java, design patterns are reusable solutions to common programming challenges. They are meant to assist developers in writing more manageable and readable code.
A singleton design pattern is a creational design pattern used to limit a class’s instantiation to one object. This is accomplished by declaring the class as a sealed class and use a private constructor to prohibit the creation of any additional objects.
The angular design pattern is a structural design pattern that divides an object’s tasks into discrete components, allowing each portion to be separately changed or expanded. This division of concerns enables more modular and testable programming.
A typical criticism of the singleton design pattern is that it might result in difficult-to-maintain and-test code. This is due to the tightly coupled of the singleton object to the code that utilizes it, making it difficult to update or replace the object without disrupting the code that relies on it.
When we need to assure that just one instance of a class is created, we utilize the singleton design pattern. This is helpful when just one object should have access to particular resources or data.
Yes, design patterns are still relevant. They are an effective technique for solving common design issues and for improving the design of existing programs.
There are 23 well known design patterns.
Here are some of design patterns in microservices:
Circuit Breaker
Event Sourcing
Aggregator
Decomposition
Asynchronous Messaging
API Gateway
Command Query Responsibility Segregator
Database or Shared Data
Branch
Chained or Chain of Responsibility
The most common method is to implement a Singleton by defining a standard class and ensuring that it has: A private builder. A static field with a single instance. A static factory method that returns the instance.
Yes. Dependency Injection is a design pattern, not a framework. Dependency injection is a software design approach that helps developers to eliminate hard-coded dependencies and improve the consistency of their programs.
The builder design pattern is a software design pattern that enables the creation of complex objects with particular, and often complicated, configuration possibilities. This design pattern is often used in Java applications to generate objects with many needed parameters and to enable the development of these objects without defining all of the required parameters ahead of time.
A facade design pattern is a kind of object-oriented design pattern that gives a consistent interface to a collection of subsystems. It is often used in combination with the builder pattern to offer a smooth interface for object creation.
The Factory design pattern is a creational design pattern that enables object creation to be abstracted. The Factory pattern is helpful when it is necessary to generate an object that is unknown at compile time, or when the kind of object to be created must be decided at run time.
A Saga design pattern is a design pattern that is used in a microservices architecture to maintain data consistency. Each service in a Saga has its own database, and the data is replicated throughout the services.
When designing objects with several needed fields, the builder design pattern is most commonly used. You may construct an object with all of the essential fields set by using a builder instead of setting each field separately.
When representing a part-whole hierarchy, the composite design pattern should be used. This pattern generates a class with a collection of its own objects. This class has methods for manipulating these items as a group.
The strategy design pattern is a behavioral design pattern that allows the behavior of an algorithm to be chosen at runtime. When an application has to allow various behaviors for different reasons, or when an algorithm needs to be picked at runtime depending on dynamic situations, this pattern is useful.
Design patterns provide a standardized solution to a common issue that may be used in a variety of situations. This saves valuable developers’ time to focus on building new and creative applications rather than recreating the wheel.
Design patterns give a common language for designers to communicate with one another. Designers can rapidly express solutions to common issues in a language that other designers can understand by utilizing design patterns.
Design patterns provide generic solutions to particular issues that may be used in a variety of situations. This enables for code reuse and makes developing new software systems faster.
Here are some step-by-step instructions for implementing design patterns:
- For an overview, read the pattern once.
- Review the sections on Structure,
- Participants, and Collaborations.
A concrete sample of the pattern code may be seen in the Source Code section. - Choose names for pattern participants that are relevant in the context of the application.
- Determine which classes already exist in your application.
- Define application-specific names for the pattern’s operations.
- Implement the operations in the pattern to carry out the duties and collaborations.
There are many approaches of breaking the singleton design pattern:
- You may break the pattern by executing the private constructor of the Singleton class through reflection.
- You may serialize the Singleton class and save it to a file using serialization. When you deserialize the class, a new instance is produced, which breaks the pattern.
- You may break the pattern by creating two or more instances of the Singleton class in a multi-threaded environment.
The first step is to determine if the issue is essentially creational, structural, or behavioral in origin. Once you’ve determined which design is most suited to your situation, it’s time to determine which ones are best suited to your individual needs.
Singleton Design Pattern
The Singleton design pattern is a software design pattern that restricts an object’s instantiation to a single instance. The pattern restricts the number of instantiations of the same class to a single instance. In addition, singleton classes are more efficient to develop and maintain.
A singleton is defined by a static attribute and a private or public accessor function. The Singleton creates an instance only when one is required. This design pattern is very useful when global variables cannot be used to create a single instance. It also provides a number of benefits over global variables.
The Singleton pattern is useful because it allows for a single point of access for all objects in the system. This helps prevent unwanted objects from being created. It also supports early and lazy instantiation, which saves memory. This design pattern is most commonly used in database applications and multi-threaded applications. It can also be used to create configuration settings and thread pools.
Factory Design Pattern
The Factory design pattern allows you to create objects without defining the class. This pattern can be used in a number of different situations, including creating reusable assets or a custom web service. It also allows you to create multiple objects in one step, so you can start testing them out in less time. This pattern is extremely flexible and can work in almost any situation.
This design pattern can solve a variety of serialization issues, especially with music. The concrete implementation of an interface is determined by a separate component. A new method called _serialize_to_xml() determines which concrete implementation to use. The new method must evaluate the value of the format parameter and return its result.
The Factory design pattern saves system resources. In the example above, the factory creates a family of related objects. For example, a furniture factory could produce a collection of different models. Creating a family of furniture is a common scenario, and customers can get very angry when they cannot find the exact same item from a catalog.
Facade Design Pattern
The Facade design pattern is a popular design pattern used in software development. The name comes from the analogy of architecture: a facade serves as a front-facing interface, while the more complex underlying code is hidden behind it. The Facade pattern is useful for many reasons, including simplicity, readability, and code reuse.
The Facade design pattern simplifies complex subsystem interfaces to provide an easy-to-use interface to the general public. It can also simplify a complex system by encapsulating the entire subsystem in a single interface object. This reduces the learning curve and promotes decoupling from clients. Using a facade can be a useful design pattern when you want your application to integrate with sophisticated libraries.
The Facade design pattern is similar to the Adapter pattern, but it simplifies the design of the interface and reduces the complexity of the subsystems. Both patterns have the same goal: to create a simple interface for the user and reduce API complexity.
MVC Design Pattern
The MVC design pattern is an architectural design style that separates program logic into three interrelated parts: the model, the view, and the controller. The model is responsible for internal representations of information, while the view is responsible for how information is displayed to a user. Using this design style allows you to create user interfaces that are both efficient and flexible.
This design pattern is useful for web applications. It allows two separate teams to work on an application, one on the backend and the other on the frontend. Developers on the backend focus on the server-side logic, while front-end developers focus on the user interface. It also avoids the’spiral’ effect of programming a complex program with many separate components.
In a typical application, each controller responds to the views. It monitors the user’s input and sends instructions to the model to maintain the data. A good example of a model is a restaurant. Customers wait for a waiter to arrive, who then records the information and moves to the kitchen. The waiter, however, does not prepare the food.
Strategy Design Pattern
The Strategy design pattern is an important pattern in Java. It allows developers to define a family of algorithms that can be used interchangeably to build applications. For example, in a game where a player has to run around a circuit and throw projectiles at targets, one might use the Strategy design pattern to specify how many hits the player needs to score to earn points. The strategy design pattern can also be used to implement other key principles of Java such as Single Responsibility Principle and Explicit Dependencies Principle. Moreover, this design pattern is important in the context of Inversion of Control Containers and Dependency Injection.
Another key concept of the Strategy pattern is its ability to eliminate conditional logic. To implement this design pattern, developers need to create classes containing the algorithms that they plan to use. Then, they need to reference these classes in their main classes. This way, when a client requests a particular strategy, the context does not have to determine which algorithm to use. The client will pass the desired strategy to the context.

Java Design Pattern
The java design pattern is a language-independent design strategy that solves common problems in object-oriented programming. Its benefits are that it is easy to understand and use by a software engineer, and it is adaptable to the needs of the application. It also allows for quick monitoring and modification. This makes it a valuable practice for IT professionals.
A common example of a design pattern is the decorator pattern. This is a style of code that encapsulates a command request as an object and allows it to be re-used by a different class or object. It enables the user to add or remove behavior at runtime, and it does so without causing problems for other objects of the same class.
Another common design pattern is the composite pattern. This design pattern lets you treat part-objects and whole objects similarly. It also makes it easy to add different kinds of components to the same application. The client code does not know the difference between the two types of objects, and the composite class implements the same interface as the individual parts.
Observer Design Pattern
The Observer design pattern defines a one-to-many dependency between objects, such as a subject and an observer. The Observer will keep track of the state of a subject, and will notify all other objects when it changes. Observer classes must implement an update method, which reacts to changes in the state of a subject.
Observer design patterns are often used in systems that contain a large number of objects. For example, an eCommerce site may send daily newsletters to subscribers. One of these subscribers may decide to unsubscribe, but thousands of others may be doing the same thing at the same time. The Observer pattern provides a scalable and proven design for these types of applications.
The Observer design pattern allows a single class to be implemented by a variety of abstractions. One type of abstract class is an ObserverBase. This class contains all observers. Each observer implements a method that is called by the subject whenever its state changes.
Adapter Design Pattern
The Adapter design pattern is used to link two classes. For example, a client class may require that a function return the result of a query in a specific format, but the adapter must first convert the response into a format that the client will understand. In such cases, the adapter class can use composition and delegation to provide a link between the two classes.
Adapters enable you to reuse existing code by wrapping legacy components in a new interface. This can be an excellent option when off-the-shelf components provide compelling functionality but are not compatible with the system architecture. Additionally, the Adapter strategy can be implemented using inheritance and aggregation. In other words, an adapter wraps an existing class with a new interface that allows clients to call methods.
The adapter design pattern is one of the Gang of Four design patterns that helps developers solve recurring problems. It lets you create a reusable and flexible application by converting two incompatible interfaces into a single one. This pattern also allows you to retrofit it to an existing object hierarchy.