Data Structures Graphs 2023

A data structure is a type of storage used to organize and store data. It is a method of organizing data on a computer so that it may be easily accessible and modified. Data structures are created to help the computer make sense of the information. They have a particular organizing principle and perform functions such as search, sorting, or aggregation (collecting data). A few decades ago, computers used sequential access memory for data storage. Different data types would be stored at different addresses in the same memory region. Before every piece of data could be accessed, it had to go through a series of steps, and all other bits had to be searched through at random. Data structures were created that helped speed up processes and enable greater automation using machine learning algorithms on modern computers with random-access memory.
A data structure is a specified format in computer programming for efficiently storing, accessing, and processing data in a computer program. Some data structures are pre-installed in programming languages, while others may require the installation of a library or module. They can be built for many different reasons, but data structures generally fall into either an abstract data type or concrete data. Abstract data types are objects that have an operation or set of operations to input and output data. At the same time, concrete datatypes are the actual realization of these operations, such as using a linked list or binary search tree to store and retrieve information. An algorithm is usually needed to convert between representations, although this is not always the case with purely abstract data types.
Free Data Structures Practice Test Online
Sustainable Data Structures
A sustainable data structure is a type of data structure that allows for the long-term storage of information, with or without an electrical or electronic power supply. Technologies used include magnetic storage media like hard disks and memory chips, as well as other types such as optical discs.
A few data structures like a list allow random access, where an element can be accessed in O(1) time (or constant time). These are said to be “random access because all the elements can be stored on a disk with no pointers, allowing for direct “random” access. In contrast, arrays do not allow random access; in order to locate an element in the array, it must be moved sequentially from its start address until it is found.
Runtime Data Structures
At runtime, efficient and cacheable C++ data structures are used. Their data-oriented architecture aims to organize them (memory-wise) based on how they’re used in real-time, maximizing CPU capabilities while minimizing memory footprint. All data accessors in the Ozz-animation runtime are const, and data structures in the runtime cannot be altered programmatically. The Ozz-animation runtime defines global data structures that combine global and local memory representations. This means that as long as the dimensions match, the same type (vector/matrix) can be represented in both global and local memory. Vectors and matrices have a fixed 8-byte alignment in any instance to ensure efficient CPU cache use and a small memory footprint.
Advanced Data Structures Application
Data Structures are used to store and organize data so that it may be accessed and modified more quickly and easily. Examples of basic data structures include Arrays, LinkedList, Stacks, Queues, and more. CubeMaps and the Red-Black Tree are examples of sophisticated data structures.
Data Structures in R
Data structures are used to store many values in R programming. Some of the most common data structures used in R include vectors, lists, matrices, data frames, and factors. We rarely interact with data that has just one value, such as a single number, such as 23, or a single word or phrase, such as “twenty-three,” since data structures are designed to accommodate multiple values. R employs a variety of data structures. R structures include scalars, vectors, matrices, arrays, data frames, and lists.
Embedded Systems Data Structures
An Embedded System contains many different data structures that are used to store data required to perform necessary tasks. They are generally made up of registers, which are used as a temporary storage location, and memory locations in the form of RAM or ROM. The register data structure is used in every embedded system since it is the fastest memory to retrieve from and write to. The program counter (PC) tells the CPU where to find the next instruction, but it also contains a few other important pieces of information, such as status flags and the interrupt level that need attention.
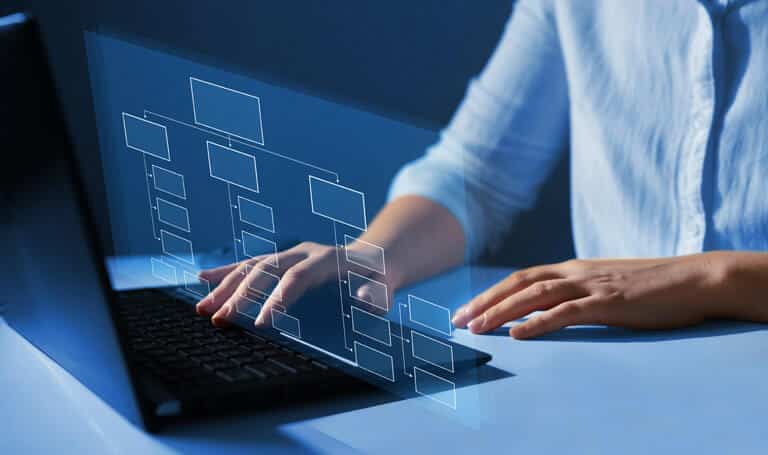
Advanced Algorithms and Data Structures
Advanced Algorithms and Data Structures cover a wide range of algorithms for complex programming problems in data analysis, machine learning, and graph computing. You’ll discover cutting-edge solutions to a variety of problems.
Advanced Algorithms and Data Structures give you effective solutions to a variety of difficult coding problems that you can adapt and use in your own applications. This practical guide updates your programming arsenal with new insights and hands-on skills by providing a balanced blend of traditional, advanced, and novel algorithms. Advanced Algorithms and Data Structures give you practical tips, tricks, and techniques for using algorithms to solve challenging problems. You’ll learn about the more recent developments that have made data mining more powerful with the introduction of machine learning algorithms.
Data Structures Advantages and Disadvantages
Each data format has its own set of benefits and drawbacks.
- The advantages of data structure
The data structure enhances the storage device’s data storage efficiency. Its use of structure facilitates data retrieval from a storage media. It can successfully and efficiently handle both little and large amounts of data. The majority of well-organized data structures, such as arrays, stacks, queues, graphs, trees, and linked lists, have well-built and pre-planned techniques for storage, retrieval, manipulation, and deletion. When employing these data structures, the programmer can completely trust them. - Data structure’s disadvantages
Using complex data structures to manage a huge amount of data can impose a high overhead on the CPU. This is because, in order to access any piece of data, a lot of work is done by the CPU. The more complex the structure is, the more overhead will be imposed on the CPU, and thus, memory consumption increases as well. The size of space required in memory can be beyond our calculations if we are not careful in choosing appropriate data structures and algorithms to deal with data.
Polymorphic Data Structures
Polymorphic data structures are required, as the system would like to have the same interface for each variant in a managed collection. When querying for documents by their schema, the storage of documents’ fields is done in a manner that is consistent across all variants. This type of data structure can be implemented using the polymorphic data type (PDT).
Polymorphic data structures are easier to implement than non-polymorphic ones. The storage mechanism for storing these types of data structures is simpler since there are only two types of values that need to be stored, i.e., instances and instances of the class descriptor. When storing a new instance into the data structure, a factory function returns the correct descriptor class; then, it is up to this class to store the actual data.
Scala Data Structures Implementation
A Scala DataTable is a small, in-memory table structure created in the Scala programming language. The implementation is unchangeable. Any changes to the table, such as adding or removing columns, rows, or individual field values, will result in a new structure being created and returned, leaving the previous one unaffected. Because of structural sharing, this is highly efficient. This makes the table extremely flexible and reusable, as only the table structure needs to be changed; the contents of each item are not touched.
Static vs Dynamic Data Structures
The size of a static data structure is predetermined. The data structure’s content can be changed without affecting the memory space allotted to it. Static data structures have been designed for specific applications, therefore have fewer resizing problems, and can be used for a longer period. When a static data structure is resized, it will just rearrange its contents within itself. Â
The size of a dynamic data structure, on the other hand, can be changed at any time. Dynamic data types are usually created by programmers to handle certain application scenarios and are not designed with resizing in mind. Dynamic data structures suffer from the disadvantage of their content not being fixed at runtime. On the other hand, this means that dynamic structures tend to be simple, whereas static types may be more complex.
Data Structures Arduino
The Arduino treats data structures like a single memory block. When you create a data structure, the Arduino creates a memory reservation based on all of the data elements in the data structure. Creating, storing, and accessing data structures is a straightforward process that requires little coding. Your application creates a temporary data structure that is stored in Arduino’s global memory. The data structure only contains a single copy of each element, so this version of the data structure contains all of the elements. When you access an item, the Arduino copies an element from its own memory block into that place within your application’s memory block.
The Arduino automatically resizes its internal blocks when needed, storing only as much data as necessary within each block in the form of contiguous bytes (as opposed to very large objects). Each time you want to store another item, you just add one more byte to that same address in the corresponding piece of global memory.
Visual Representation of Data Structures
The data structures can be visualized in different ways to give a better understanding of the data structure’s internal representation. Here are some visual representations of different data structures:
Some of these representations are created by using highlighting and color-coding. By using three colors to represent values in a table, blue represents integer values, green represents null, and red represents boolean values. Other representations have been designed with the help of graphs, flow charts, or other diagrammatic forms. By using these types of representations, we can get a better understanding of the structure’s working principles and relationships between its parts.
Data Structures and Abstractions with Java
Data abstraction is a completely separate animal. It is used in OOP to unify all the generic and most common attributes of a data structure in order to establish a foundation for your software architecture and avoid redundancy.
The term “abstraction” refers to displaying only the information that the intended user needs. Abstraction is the “abstract form of anything,” as the term implies. The abstract form of a data structure or underlying representation of the data structure.
In other words, data abstraction is used to depict any and all details about a data structure by using only non-abstract attributes such as position, size, shape, or some other abstract concept. This will allow anyone to read or use the visual representation of this abstraction without knowing how the information represented really got into that form in the first place. In general, it is best to make a model very simple in order to keep complexity at a minimum.
Lock Free Data Structures
To boost performance, utilize a lock-free data structure. Because access to the shared data structure does not need to be serialized to stay coherent, a lock-free data structure increases the amount of time spent in parallel execution rather than serial execution, enhancing performance on a multi-core processor.
Concurrent data structures are data structures that allow for concurrency: multiple processes or threads can safely access the element at the same time. Normally, all actions are serialized (that is, nothing else is allowed to access the object until the first action has ended). The main problem with this approach is that it might lead to a race condition. Without a lock-free data structure, there is no efficient way to wait for another thread to finish its task without blocking the original thread. This may have unintended side effects as well: if one of the threads allocates a resource (like memory) while another thread needs the same resource, then a deadlock might occur. Concurrent data structures prevent these problems by using different synchronization techniques.
Data Structures Questions and Answers
An array is a linear data structure that stores components of the same data type in memory places that are contiguous and adjacent. Arrays use an index system that runs from 0 to (n-1), where n is the array’s size.
A data structure is a method of organizing data in a computer so that it may be efficiently used.
A tree is a hierarchical, non-linear data structure made up of numerous nodes connected by edges. A tree is an abstract data type (ADT) that collects data in a hierarchical fashion.
The following are the several types of data structures in Java.
- a list of links
- Arrays
- Queue
- Set
- Arrays
- set
Unstructured data is typically stored in its original format, but structured data is clearly defined and searchable. Quantitative data is structured, but qualitative data is unstructured. Data warehouses are used to store structured data, and data lakes are used to store unstructured data.
When it’s required to repeatedly delete the object with the highest (or lowest) priority, or when insertions must be interspersed with removals of the root node, a heap is a useful data structure. The binary heap, in which the tree is a binary tree, is a typical implementation of a heap.
Breadth-First Search stores the node and marks it as “visited” using a queue data structure until it has marked all of the nearby vertices directly connected to it. Because the queue follows the First In First Out (FIFO) principle, the node’s neighbors will be shown in the order in which they were entered into the node, starting with the first node.
It’s a linear data structure with insertion and deletion at the back end and front end, respectively.
Heap is a type of balanced binary tree data structure in which the root-node key is compared to its children and then sorted.
Spreadsheets, in addition to relational databases, are common sources of structured data. Because structured data relies on you building a data model, you must plan for how you will capture, store, and retrieve data, whether it’s a complex SQL database or an Excel spreadsheet.
A Heap is a special type of Tree-based data structure in which the tree is a full binary tree.
The system’s primitive, or building block, is the spatial data structure. There will be a limit to the number of different types of spatial data structures that can be used.
A bag is a collection of objects to which you can continue to add objects, but you cannot remove them after they have been added to the bag. So, using a bag data structure, you can collect all of the items and then iterate over them. When you program in Java, you will generally get a bag.
An ADT is a way of looking at a data structure. A computer programmer uses abstraction to focus on what it does rather than how it does its work in order to deal with difficult challenges. ADTs are entirely theoretical entities that are used to ease the description of algorithms and to categorize and assess data structures.
Immutable data structures are data structures that cannot be altered, such as lists, arrays, and sets. This means that the values inside them can not be added, removed, moved, or exchanged. Instead of modifying the data structure, you create a new version of the data structure that is a separate value.
Data and one or more linkages to other nodes are stored in nodes, which are the most fundamental data structure. Nodes can be represented by tree structures or linked lists. It is possible to traverse from one node to another in such structures that use nodes. Orphaned nodes are those that have no links connecting them except the head node.
A comprehensive implementation of the graph data structure is not available in Java. Collections in Java, on the other hand, can be used to programmatically represent the graph. We can also use dynamic arrays like vectors to create graphs. In most cases, we use the HashMap collection to implement graphs in Java.
R does not contain any scalars because vectorized operations are so crucial in R. A vector, or a sequence of data objects, is the most fundamental data structure. As a result, a single integer value is regarded as a unit-length integer vector.
Semi-structured files are CSV files. Semistructured data lacks the same level of organization as structured data, such as that found in relational databases. The data contains elements that can be used to divide the data into different hierarchies.
A hashmap is a data structure capable of mapping specific keys to specific values. It doesn’t matter what the keys and values are.
A hash table is a key-value pair storage data structure. Hash tables employ the hash function to find an index into an array where an element can be inserted or searched for. In open-addressed hash tables, linear probing is a method for resolving collisions.
Kernel data structures are crucial because they hold information about the system’s present state. The kernel and its subsystems have exclusive access to the majority of kernel data structures.
The most basic R data objects are vectors, which are the most basic data structures in R. Atomic vectors are divided into six categories: logical, integer, character, double, and raw. “A vector is a group of elements that are most typical of the character, integer, logical, or numeric types.”
Computer programmers use LIFO and FIFO terms to describe how data is handled, or the data structure. Data must be retrieved in a variety of ways, whether randomly or sequentially, for various processes. Stacks and queues are two types of sequential data structures that follow the LIFO and FIFO concepts, respectively.
When the values in a sorted array are uniformly distributed, interpolation search is a better option than binary search. To check, binary search always goes to the middle element. Interpolation searching, on the other hand, may lead to different places depending on the value of the key being searched.
Lock-Free: A data structure is lock-free if at least one thread can execute it at any given time. It’s possible that all of the other threads are starved. The distinction between obstruction-freedom and obstruction-freedom is that even if no threads are suspended, there is at least one non-starving thread.
Data that is neither captured nor formatted in a traditional way is referred to as semi-structured data. Because it lacks a fixed schema, semi-structured data does not adhere to the same format as tabular data or relational databases.
In programming, the abstract data structure “dictionary” (Associative Array, Map) is represented by multiple aggregated pairs (key, value) together with established methods for retrieving the values by a particular key. This data structure is sometimes known as a “map” or “associative array.”
Data structures are used to improve an application’s efficiency and performance by organizing data in such a way that it takes up less space while processing at a faster rate.
Python is an excellent language for learning algorithms and data structures because it has a simple, clear syntax that resembles pseudocode. The language’s simplicity allows you to concentrate on creating the algorithm rather than the syntax. Looking at Python code makes it much easier to comprehend how an algorithm works than looking at code written in any other language.
Implementing a data structure or algorithm is one of the best ways to learn about it. While reading about distinct chaining hash tables is one thing, actually having to create the code will give you a far better grasp of how they work.
A data structure is a method of organizing data on a computer so that it may be efficiently used.
Data structures and algorithms are significant for a variety of reasons, including the fact that they can help you find new jobs and improve your skills as an engineer and problem solver.
Algorithms and data structures are inextricably linked. You’ll choose a problem to solve and try to put a solution in place. If you’re familiar with algorithms, you’ll know that they’re nothing more than a method for solving a problem in a systematic fashion.
A trie is a discrete data structure that isn’t well-known or discussed in standard algorithm classes but is nonetheless important.
A DAG is a computer science data structure that can be used to simulate a wide range of situations.
Sorting is the process of arranging or placing a collection’s parts in some sort of order. It simply refers to the storage of data in a sorted sequence. It is possible to sort in both ascending and descending order. It organizes the data in a logical order to facilitate searching.
The entire memory space required by the program for its execution is known as its space complexity. Worst Case Time Complexity of various data structures for various operations Average Time Complexity of various data structures for various operations
TRIE is the best data format for searching strings quickly.
With appropriate materials and coaching, Data Structures and Algorithms can be studied in 6–12 months, depending on the individual’s learning potential for this topic and other influencing circumstances. Data Structures and Algorithms are a constantly evolving field of study, and achieving absolute efficiency can take a lifetime.
Data should be imported. List (maximum) and Data.Tree (built-in tree data structure). Define a function to compute the height of a tree: height: Tree a-> Int height (Node val []) = 1 height (Node val xs) = 1 + maximum (map height xs)
Overall, data structures are a simple class to learn. However, there is a lot to learn, it is a crucial lesson, and it has some challenging parts. There are several things that will influence how challenging the lesson is for you. There are, however, a few things you can do to make it a lot easier.
Unstructured data can include anything, including film, imaging, music, sensor data, text data, and much more. Datasets (often big collections of files) that aren’t stored in a structured database format are referred to as unstructured.
In Python, data structures and algorithms are two of the most basic computer science topics. For any programmer, these are essential tools. In Python, data structures are used to organize and store data in memory while a program is running.