ASP NET Core Web API 2023
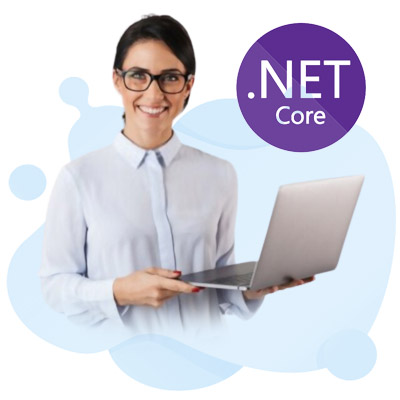
ASP.NET Core is a new open-source, cross-platform framework for developing modern cloud-based internet-connected applications like web, IoT, and mobile backends. Apps written in ASP.NET Core can run on either.NET Core or the full.NET Framework. It was designed to provide an optimized development framework for programs that run on-premises or in the cloud. It’s modular components with low overhead, giving you more options when building solutions. Your ASP.NET Core apps can be developed and operated on Windows, Mac, and Linux. The source code for ASP.NET Core is available on GitHub. ASP.NET Core is an evolution of ASP.NET, which first appeared in January 2002, during the dot-com bubble. The initial release of ASP.NET was a free package for web development on Windows servers. Today, there are three major versions of ASP.NET
The architectural improvements in ASP.NET Core result in a significantly leaner and modular framework. The system is no longer used in ASP.NET Core. Web.dll. It’s built on a foundation of well-factored NuGet packages. It lets you optimize your program by including the NuGet packages you require. Tighter security, reduced servicing, improved performance, and lower expenses in a pay-for-what-you-use model are advantages of a smaller app surface area. The ASP.NET Core team designed their framework specifically to create cloud-ready apps that run anywhere. They wanted to create a framework that developers and IT administrators could use with confidence, even if they didn’t know how to write code themselves.
ASP.NET Core 1.0 was released in December 2016, with the launch of Visual Studio 2017 not long after. That same month, Microsoft announced an SDK for .NET Standard 2.0, which enables cross-platform apps written in C#/VB or JavaScript for all major platforms:
- Desktop, mobile, and IoT devices via browsers and client apps such as iOS and Android apps
- Windows 10, Windows Server, or SQL Server applications using a Microsoft Graph API
- Web applications running on the Kestrel webserver
Free ASP.NET Core Practice Online Test
ASP.NET Core validation DropdownList
The ASP.NET Core Dropdown List can be used to quickly replace HTML select tags. It has a rich design and lets users choose from a list of predetermined values a single non-editable value. Data binding, filtering, grouping, UI customization, accessibility, and preselected values are all included out of the box. The DropdownList is a flexible, easy to use control and can be used in many different scenarios. For example, you can use it to populate a drop-down or text box. Just bind an object property to the DataSource property of the DropdownList, and then set the SelectedValue to the desired value in your view model.
There are two ways to validate a DropDownList value:
1. Validation Rules
By adding validation properties to the input element, validation rules assist you in verifying the selected text. The ValidationRules property can be used to set this. You may use any of the built-in validation rules or create your own.
2. Messages of Validation
The ValidationMessage property allows you to create your own unique error message. Specify the corresponding annotation attribute followed by the message to display the error message.
The DropdownList allows you to bind an object property to the DataSource property of the DropDownList, and after that, set the SelectedValue to a value in your view model. If not set prior, the selected value will be initialized by default with a default option ”.
ASP.NET Core allowedhosts
allowedHosts is used to bind your app to specific hostnames using host filtering. It is used to run your app on a specific port.
ASP.NET Core MVC will listen for incoming requests on all interfaces, using port number 5000 by default. But you can use the HostName property to limit your app’s listening interface or port number. You can use allowedHosts in conjunction with host-based routing to route requests based on the client’s address, user identity, or server name.
ASP.NET Core drag and drop
The Microsoft ASP.NET Core MVC Drag and Drop widget can help you add drag-and-drop functionality to your modern and intuitive application. The widget works best with the handler attribute on your data-bound controls, which allows you to programmatically determine when the user has started dragging data from a source into your control.
You can use the attribute anytime during your application process, including within data binding expressions or even in your controller action. You can also dynamically change the dragged object using an embedded ViewModel and methods such as SetDragImage and SetLiftedImage. You’ll be able to use the new ASP.NET Core drag and drop functionality by moving an image from one side of your application to the other. You first bind the source image tag in your view to your source property in your model.
When you move your mouse over it, a cursor change occurs, and you may use the handler attribute to link this event to a method on your controller. This function is called as soon as you start dragging an object from one side of your application to another, providing both the source and target objects for you to perform some custom logic when an object is being dragged around.
When dropping an object, two events are raised: DragDropEnd and DragDropStarted.
ASP.NET Core FromForm
The FromForm element is for incoming data from a submitted form sent with the content type application/x-www-form-urlencoded, whereas the FromBody attribute parses the model from the request body in the default fashion, which is usually sent with the content-type application/json. The FromForm attribute specifies that the data for a model is obtained from a form field.
You can easily bind the form field names for the data source to your model properties. You also need to set up content negotiation to tell ASP.NET MVC how to parse incoming requests as form content, JSON, or XML. You can use the attribute on your controller action or directly on your model property. However, it’s generally best to place it on a controller action if you’re using multiple actions per controller.
The basic idea behind this element is that you have one parameter whose value contains several parameters automatically forwarded to your model object as strongly typed parameter values using data binding.
ASP.NET Core MariaDB
One of the most popular open-source relational databases is MariaDB Server. It was created by the original MySQL developers and is promised to remain open source. Most cloud providers use it, and most Linux distributions use it by default. Therefore, it can be considered a default choice for developers. You can easily use Microsoft’s Entity Framework Core to utilize MariaDB’s full support for SQL Standards compliance easily. The protocol is based on the open standards JDBC, SQL/CLI, and ODBC.
ASP.NET Core message queue
Message queues provide asynchronous communication and processing between different portions of a system. We can separate the operation, disconnect the services, and scale independently in this fashion. We can handle huge loads and distribute the system’s components without hindering performance or availability. You can use message queues to communicate within a web application, across different services, and even external systems.
The message queue is a centralized container for messages delivered to recipients in a predefined sequence. In other words, you put data in a queue, and it’s then processed by some consumer later, asynchronously. A popular choice for ASP.NET Core is RabbitMQ.
ASP.NET Core server side rendering
Server-Side Rendering (SSR) is a technique used to prerender and cache the page content on the server-side when a request comes in. In other words, you can use it to process the page at the server-side after receiving the first request and send back HTML markup as a response. You can use it to implement a demo or a working application. You need to include the script tag that contains the markup of the page you want to prerender and cache.
The capacity of an application to turn HTML files on the server into a fully rendered HTML page for the client is known as server-side rendering (SSR). The web browser sends a request for information to the server, answering immediately by delivering a completely displayed page to the client. This approach is a good choice for small, simple applications that don’t require a lot of HTML markup. For example, you might use server-side rendering when you’re using lightweight templates instead of JavaScript frameworks like Angular: they are less demanding on your server resources because they don’t render much content.
The primary idea behind SSR is that the same page may be provided to several clients without having to be rendered on each one. Using a virtual function called prerendering, we can prerender and cache the page content on the server before sending the first request to our client application. This happens behind the scenes and doesn’t require us to modify any code.

Chart JS ASP.NET Core
With Chart.js, getting started is simple. To render the chart, all you need is the script included in your page and a single <canvas> node. Chart.js is suitable for fast data visualization, and it’s highly customizable.
You can include the script file on your page, specify the parameters for your chart (type, data, options), and draw it all with a single method, .render().
The chart is a powerful JavaScript library that makes interactive charts on the web easy. It’s simple, flexible, and fun to use. You can create anything from simple line charts and bar charts to complex geographic maps or real-time stock charts. Chart supports responsive design, so you can easily adapt it to any screen size without sacrificing quality or performance. The library is compatible with any CMS framework and offers thousands of customization possibilities.
Datatable ASP.NET Core
The DataTable is a key component of the ADO.NET framework. The DataSet and the DataView are two further objects that use the DataTable. The DataTable contains columns, which can be of different types, including ADO.NET data types (such as string, int, or binary) and HTML entities.
A key feature of DataTables is the ability to add and remove properties from these tables at runtime (pagination). You can filter data based on specific criteria using an SQL-like syntax (select clause).
Responsecache ASP.NET Core
Response caching headers are set using the ResponseCache property. The HTTP 1.1 Caching standard requires clients and intermediate proxies to respect headers while caching replies. Response caching can reduce bandwidth usage and speed up delivery to clients by providing a local copy of frequently requested resources. ResponseCache uses the output from a standard HTML compression algorithm to optimize performance. It compresses the response content with GZIP and sends compressed data to the client.
RespondCache is an advanced server-side cache library that leverages ASP.NET Core’s built-in Caching framework to add caching capabilities to your application more easily. It’ll become handy when you need to cache responses from your web API, for example, and improve its performance by providing a local copy of frequently requested resources.
On ASP, Response Caching is also possible.
Scripts render ASP.NET Core
Scripts are very useful for implementing custom logic without coding. They automate tasks, render forms, generate content, and so on. .NET provides a ScriptManager class to facilitate access from views in an ASP.NET Core app by allowing you to inject Scripts into your page. Using .NET Core 2.0, you can use Visual Studio 2017 and the Razor syntax to create your scripts for page rendering and page navigation; otherwise, you can use the Script editor that ships with Visual Studio 2017.
View Components in ASP.NET Core MVC
View components in ASP.NET Core MVC are comparable to partial views, but they are far more powerful. View components don’t use model binding. Thus they rely only on the data you provide when calling them. It allows you to create reusable components that don’t depend on the model context.
As a simple example, you could use a ViewComponent to build an admin page that is rendered once and then cached. These help significantly reduce the amount of time it takes for users to see the page and reduce pressure on your back-end application.
The reason for this is that each ViewComponent has its independent lifecycle, which means that we can dispose of every control right after its execution and cleanup resources.
In contrast, Razor views are part of the global view state, and thus they keep all their resources in memory until they are released at the end of processing the request or until specific events occur (such as exceptions being thrown).
AdminLTE ASP.NET Core
The best open source admin dashboard and control panel theme is AdminLTE. The UI framework Bootstrap was utilized. AdminLTE comes with several responsive, reusable, and widely used components. These components are ready to use with your data and can easily be customized.
AdminLTE is a ready-made, open-source, Bootstrap 3 based admin control panel template. The jQuery and Angular versions are also available. It includes many HTML pages and CSS files that provide numerous ready-to-use components for creating web applications with ASP.NET MVC5 or ASP.NET Core 2.0, like CRUD operations with Views, Basic Login Page, or Responsive Tables and Chart Controls.
The AdminLTE template is designed for developers who want to create powerful web applications faster in an easy-to-use way with great UX/UI quality.
ASP.NET Core change port
In an ASP.NET Web API, navigate the launchSettings.json file under Properties to modify the port. The default port is 4222 (this can be changed manually on the ASP.NET Core command line without doing the update). The launchSettings.json file is the configuration file that Visual Studio uses to customize your ASP.NET Core Web API project. We add the app setting key under Package/Publish/Debug and set it to a string value representing the port number we want to use.
ASP.NET Core Questions and Answers
ASP.NET Core is a cross-platform framework for creating cloud-based applications such as web apps, IoT apps, and mobile backends that are free and open-source.
Before you deploy, double-check that WebHostBuilder is set up correctly for Kestrel and IIS.
- Save the file to a folder
- Copy the files to the IIS location you want.
- In IIS, create an application.
- Download and Install Your App!
Server. Asp.net core does not provide MapPath. Instead, you can access any actual data stored in the specified location using the IWebHostEnvironment interface.
The fundamental distinction between. NET and ASP.NET is that. NET is a software framework for developing, running, and executing applications, whereas ASP.NET is a web framework for constructing dynamic web applications. NET.
ASP.NET Core apps use the IHttpContextAccessor interface and its default implementation HttpContextAccessor to access HttpContext. IHttpContextAccessor is only required when you need access to the HttpContext within a service.
Right-click References in the Solution Explorer pane and select Add Web Reference to launch the Add Web Reference dialog box. Rename the Web reference toExcelWebService in the Web reference name box. To add a Web reference for the target Web service, click Add Reference.
The following are some basic.NET Core migration steps:
- Target.NET Framework 4.7.2 or higher in all projects you want to port. This step ensures that you can use alternate APIs for.Net Framework-specific targets when.Net Core doesn’t support a specific API.
- Make use of the.Net Portability Analyzer. This tool examines assemblies and determines whether they are.Net Core portable.
- Use the.NET API analyzer to find APIs that throw PlatformNotSupportedException on certain systems and other possible compatibility concerns.
- Use the conversion tool to convert all of your packages. config dependencies to the PackageReference format as packages. config does not function on.NET Core.
- Because.NET Core uses a different project file format than the.NET Framework; you must establish new projects for it and copy source files or use a tool to convert your old files.
- You recommend that you port your test code: Porting is an example of a major shift that can backfire if things go wrong. Porting your test project and running/testing the code is strongly recommended.
ASP.Net is a popular programming language for building websites and applications because of its speed and low cost. It’s simple to pick up and requires very little setup and resources. It is also a widely used and well-known programming language.
For ASP.NET Core, IdentityServer is an authentication server that implements the OpenID Connect (OIDC) and OAuth 2.0 standards. It’s made simple to make authenticated requests to all your applications, whether web, native, mobile, or API endpoints.
The web. config file must be present in the deployed app’s content root location (usually the app base folder).
The following are the advantages of ASP.NET Core:
- A cohesive narrative for developing web UI and APIs.
- Designed to be testable.
- Razor Pages makes it easier and more productive to code page-focused situations.
- To make a partial view, right-click on the Shared folder, then choose Add, then View.. to bring up the Add View dialog.
- Select the “Create as a partial view” checkbox in the Add New Item popup and specify a partial view name. We don’t need to employ a model for this partial view, so select Empty (no model) from the Template choice and hit the Add button. In the Shared folder, this will generate an empty partial view.
- Now, you can paste it into the _MenuBar.cshtml